Navigating with Next.js Routes
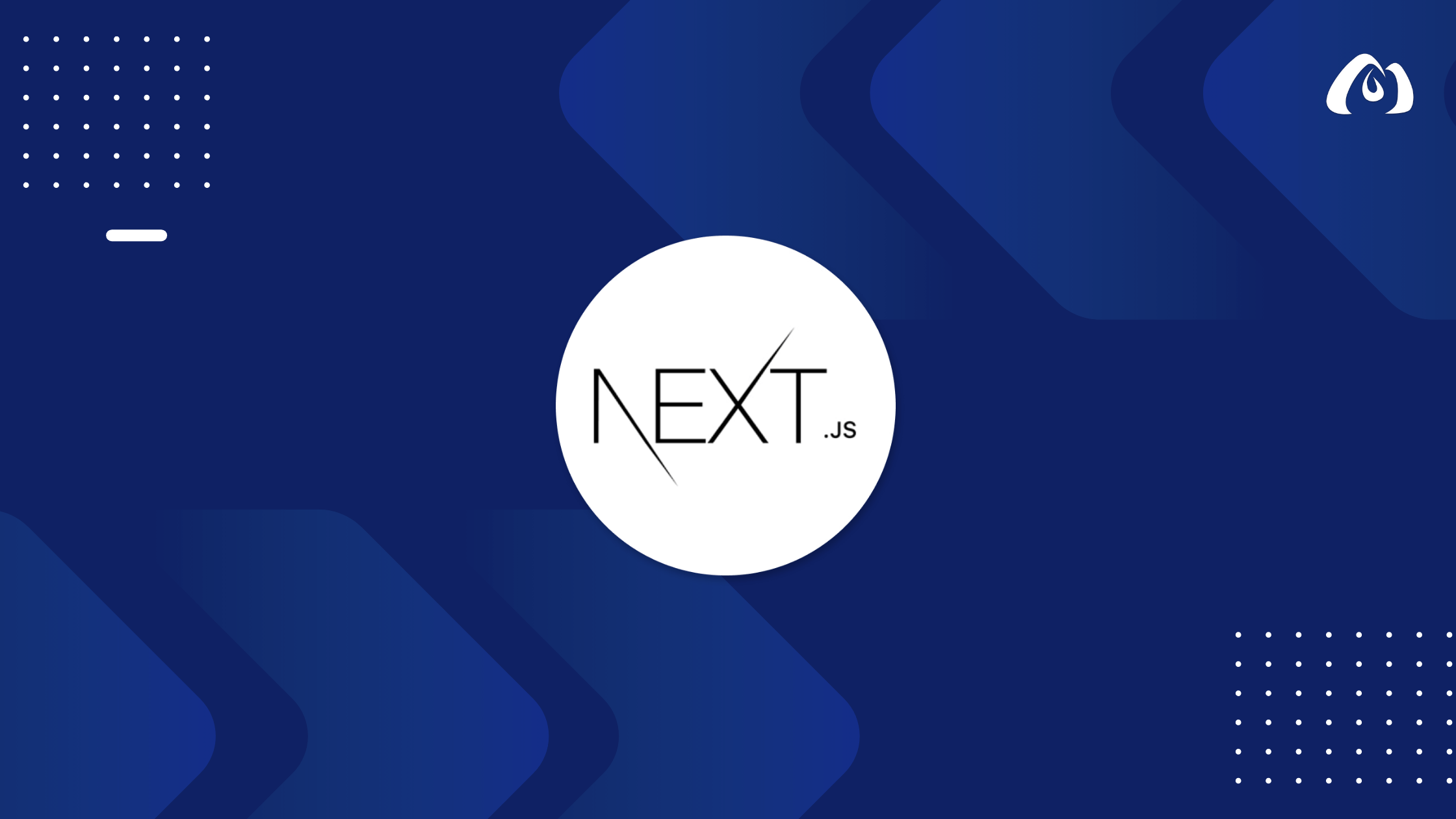
Navigation is a crucial aspect of any web application, and Next.js makes it a breeze with its powerful routing capabilities. In this tutorial, we will delve into the world of routing in Next.js, covering both static and dynamic routes.
Static Routes
Setting Up Your Next.js Project
Before we dive into routing, make sure you have a Next.js project ready. If not, you can create one using the following commands:
npx create-next-app my-next-app
cd my-next-app
Now, let’s start by creating a few static pages. Inside the pages
directory, you can add files such as about.js
and contact.js
. Each of these files represents a separate route in your application.
Creating Static Pages
In pages/about.js
:
// pages/about.js
const About = () => {
return (
<div>
<h1>About Us</h1>
<p>This is the about page of our Next.js app.</p>
</div>
);
};
export default About;
In pages/contact.js
:
// pages/contact.js
const Contact = () => {
return (
<div>
<h1>Contact Us</h1>
<p>Feel free to reach out to us!</p>
</div>
);
};
export default Contact;
Now, when you run your Next.js app (npm run dev
), you can navigate to http://localhost:3000/about
and http://localhost:3000/contact
to see the respective pages.
Dynamic Routes
Next.js allows you to create dynamic routes by using brackets []
in your page filenames.
Creating Dynamic Pages
Let’s create a dynamic page for displaying user profiles. Inside the pages
directory, create a file named [username].js
:
// pages/[username].js
const UserProfile = ({ username }) => {
return (
<div>
<h1>User Profile</h1>
<p>Welcome, {username}!</p>
</div>
);
};
export default UserProfile;
Now, you can visit http://localhost:3000/john
or http://localhost:3000/jane
to see dynamic user profiles.
Conclusion
Congratulations! You’ve learned the basics of navigation in Next.js. We covered both static and dynamic routes, allowing you to create a well-structured and dynamic web application.
In future tutorials, we’ll explore more advanced features of Next.js routing, including nested routes and route guards. Stay tuned for an exciting journey into the world of Next.js development.
Happy coding!