State Management in Next.js
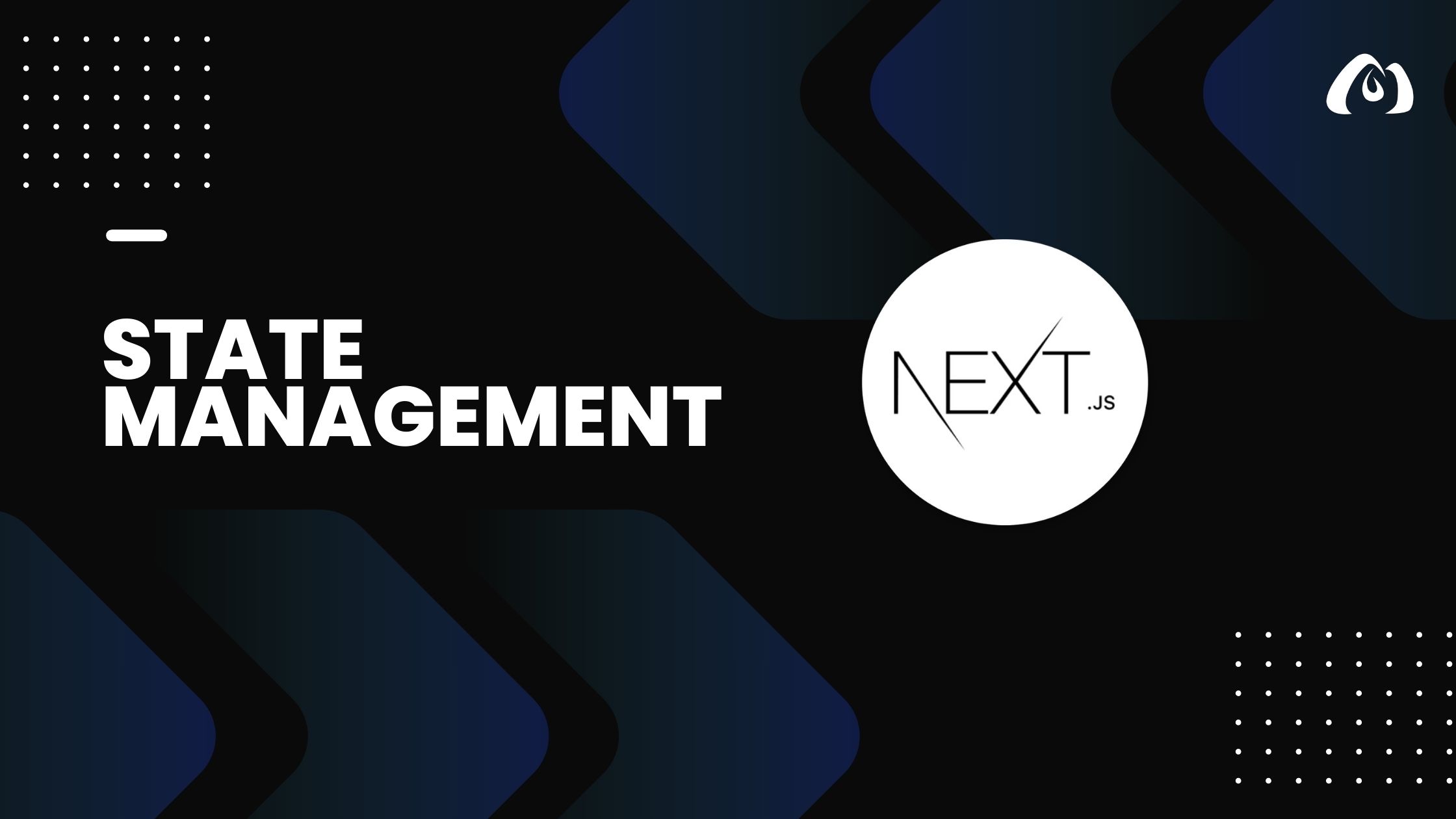
Managing state is a fundamental aspect of building React applications, and Next.js provides various options for handling state. In this tutorial, we’ll explore state management in Next.js, focusing on using the Context API for local state management within a component.
Using the Context API
The Context API is a powerful tool for managing state in a React application, allowing you to share state between components without having to pass props down through the entire component tree.
Step 1: Create a Context
Create a new file, for example, MyContext.js
, to define your context:
// MyContext.js
import { createContext, useContext, useState } from 'react';
const MyContext = createContext();
export const MyContextProvider = ({ children }) => {
const [myState, setMyState] = useState(initialState);
const updateState = newValue => {
setMyState(newValue);
};
return (
<MyContext.Provider value=>
{children}
</MyContext.Provider>
);
};
export const useMyContext = () => {
return useContext(MyContext);
};
Step 2: Wrap Your Components with the Context Provider
Wrap your top-level component or a specific section of your app with the context provider:
// pages/index.js
import { MyContextProvider } from '../path/to/MyContext';
const HomePage = () => {
return (
<MyContextProvider>
{/* Your components go here */}
</MyContextProvider>
);
};
export default HomePage;
Step 3: Use the Context in Your Components
Now, any component within the provider can access and update the shared state:
// components/MyComponent.js
import React from 'react';
import { useMyContext } from '../path/to/MyContext';
const MyComponent = () => {
const { myState, updateState } = useMyContext();
return (
<div>
<p>My State: {myState}</p>
<button onClick={() => updateState('New Value')}>Update State</button>
</div>
);
};
export default MyComponent;
Conclusion
The Context API in React is a versatile solution for local state management. While this example focuses on a simple use case, the Context API can scale to more complex applications.
In the upcoming tutorials, we’ll explore advanced topics such as user authentication, styling, and advanced routing in Next.js.
Stay tuned for the next tutorial where we’ll dive into user authentication with Next.js.
Happy coding!