User Authentication in Next.js
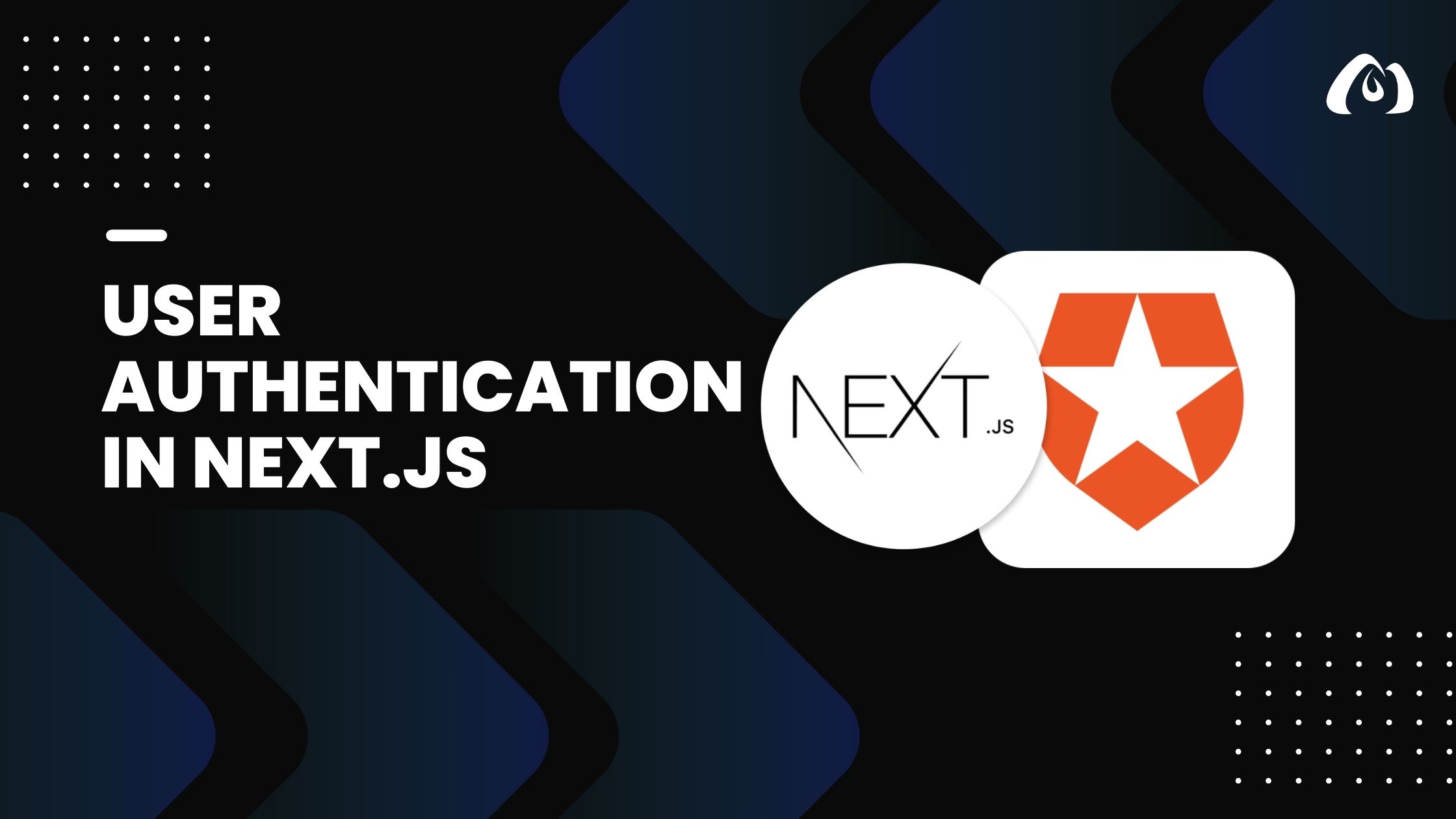
Implementing user authentication is a common requirement for web applications, and Next.js provides seamless integration with various authentication providers. In this tutorial, we’ll explore user authentication in Next.js, focusing on implementing authentication with Auth0.
Using Auth0 for User Authentication
Auth0 is a widely used authentication and authorization platform that makes it easy to add secure user authentication to your applications.
Step 1: Set Up an Auth0 Account
- Go to Auth0 and sign up for a free account.
- Create a new application in the Auth0 dashboard.
Step 2: Install Dependencies
Install the necessary packages for authentication in your Next.js app:
npm install @auth0/nextjs-auth0
Step 3: Configure Auth0
Create a file named .env.local
in your project and add the Auth0 configuration:
# .env.local
NEXT_PUBLIC_AUTH0_CLIENT_ID=your-client-id
AUTH0_CLIENT_SECRET=your-client-secret
AUTH0_BASE_URL=http://localhost:3000
AUTH0_ISSUER_BASE_URL=https://your-auth0-domain
Step 4: Implement Authentication
Create a new API route for handling authentication:
// pages/api/auth/[...nextauth].js
import NextAuth from 'next-auth';
import Providers from 'next-auth/providers';
export default NextAuth({
providers: [
Providers.Auth0({
clientId: process.env.NEXT_PUBLIC_AUTH0_CLIENT_ID,
clientSecret: process.env.AUTH0_CLIENT_SECRET,
domain: process.env.AUTH0_ISSUER_BASE_URL,
}),
],
pages: {
signIn: '/auth/signin',
signOut: '/auth/signout',
error: '/auth/error',
verifyRequest: '/auth/verify-request',
},
});
Step 5: Add Authentication to Pages
Secure pages by requiring authentication:
// pages/secure-page.js
import { useSession } from 'next-auth/react';
const SecurePage = () => {
const { data: session } = useSession();
if (!session) {
return <p>Please sign in to access this page.</p>;
}
return (
<div>
<h1>Secure Page</h1>
<p>Welcome, {session.user.name}!</p>
</div>
);
};
export default SecurePage;
Conclusion
Authenticating users in your Next.js application becomes straightforward with the Auth0 integration. This example covers a basic setup, but Auth0 provides additional features such as social logins, multi-factor authentication, and more.
In the upcoming tutorials, we’ll explore advanced topics like SEO optimization, internationalization, and testing strategies in Next.js.
Stay tuned for the next tutorial where we’ll focus on SEO optimization techniques.
Happy coding!