SEO Optimization in Next.js
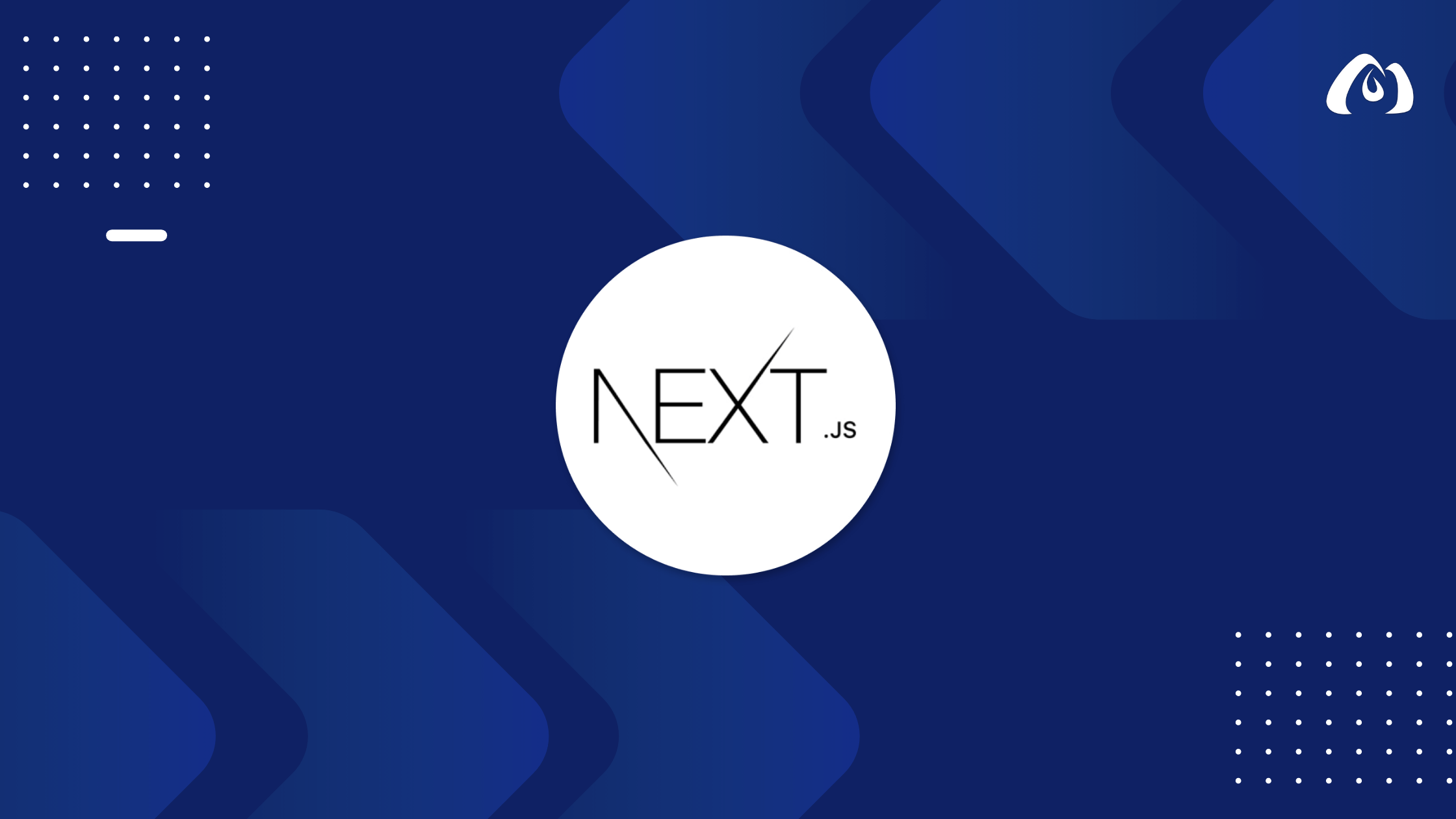
Search Engine Optimization (SEO) is crucial for ensuring your Next.js applications are discoverable by search engines. In this tutorial, we’ll explore techniques for optimizing the SEO of your Next.js projects.
1. Meta Tags with Head Component
The next/head
component allows you to modify the <head>
of your pages, making it easy to include essential meta tags.
// pages/index.js
import Head from 'next/head';
const HomePage = () => {
return (
<div>
<Head>
<title>Your Page Title</title>
<meta name="description" content="A concise description of your page." />
<meta name="robots" content="index, follow" />
</Head>
{/* Your page content goes here */}
</div>
);
};
export default HomePage;
2. Open Graph Meta Tags
Open Graph meta tags improve the appearance of your links when shared on social media platforms. Include them in your next/head
component.
// pages/index.js
import Head from 'next/head';
const HomePage = () => {
return (
<div>
<Head>
<meta property="og:title" content="Your Page Title" />
<meta property="og:description" content="A concise description of your page." />
<meta property="og:image" content="/path/to/your/image.jpg" />
<meta property="og:url" content="https://your-website.com" />
<meta name="twitter:card" content="summary_large_image" />
</Head>
{/* Your page content goes here */}
</div>
);
};
export default HomePage;
3. Sitemap and Robots.txt
Create a robots.txt
file to specify which parts of your site search engines should crawl. Also, consider generating a sitemap to help search engines understand your site structure.
4. Dynamic Page Titles
Make your page titles dynamic by using data from the page or the URL.
// pages/[slug].js
import { useRouter } from 'next/router';
import Head from 'next/head';
const DynamicPage = () => {
const router = useRouter();
const { slug } = router.query;
return (
<div>
<Head>
<title>{`Dynamic Page - ${slug}`}</title>
</Head>
{/* Your dynamic page content goes here */}
</div>
);
};
export default DynamicPage;
Conclusion
Optimizing your Next.js application for search engines is essential for reaching a wider audience. By incorporating meta tags, open graph tags, and dynamic page titles, you can enhance the SEO of your Next.js projects.
In the upcoming tutorials, we’ll delve into internationalization, testing strategies, and deployment options for Next.js.
Stay tuned for the next tutorial where we’ll explore internationalization in Next.js.
Happy coding!