Integrating Redux into Your Next.js App
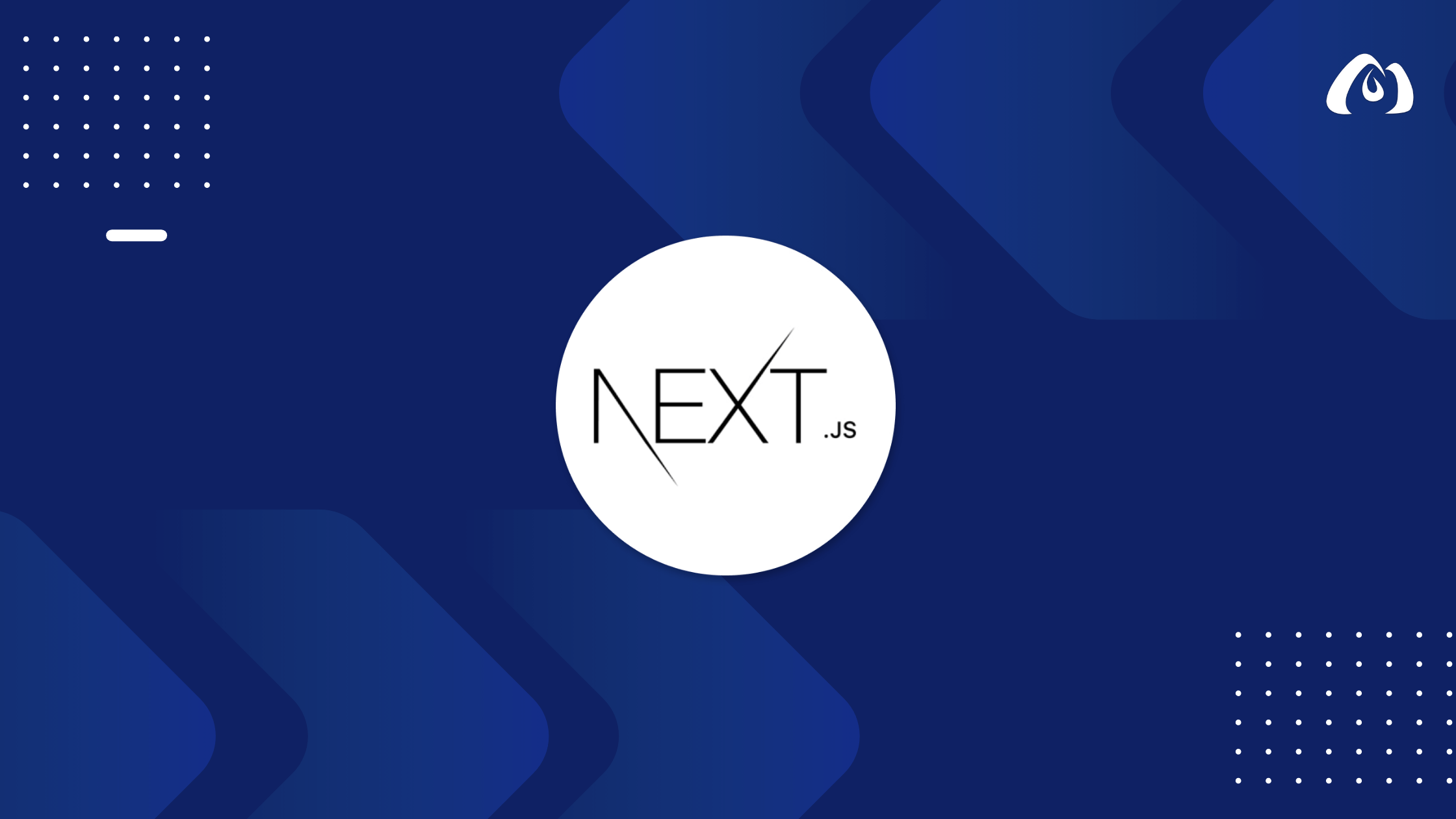
Redux is a powerful state management library that can enhance the management of your application’s state. In this tutorial, we’ll explore how to integrate Redux into your Next.js application to manage global state effectively.
Setting Up Redux in Next.js
Step 1: Install Dependencies
Start by installing the required packages:
npm install redux react-redux
Step 2: Create Redux Store
Create a store
directory in your project and set up the Redux store:
// store/index.js
import { createStore } from 'redux';
import rootReducer from './reducers';
const store = createStore(rootReducer);
export default store;
Step 3: Create Reducers
Inside the store
directory, create a reducers
directory and add a sample reducer:
// store/reducers/sampleReducer.js
const initialState = {
data: [],
};
const sampleReducer = (state = initialState, action) => {
switch (action.type) {
case 'ADD_DATA':
return { ...state, data: [...state.data, action.payload] };
default:
return state;
}
};
export default sampleReducer;
Step 4: Combine Reducers
Combine reducers in the rootReducer.js
file:
// store/reducers/rootReducer.js
import { combineReducers } from 'redux';
import sampleReducer from './sampleReducer';
const rootReducer = combineReducers({
sample: sampleReducer,
});
export default rootReducer;
Step 5: Wrap Your App with the Redux Provider
In your _app.js
or _app.tsx
file, wrap your entire application with the Redux Provider
:
// pages/_app.js
import { Provider } from 'react-redux';
import store from '../store';
function MyApp({ Component, pageProps }) {
return (
<Provider store={store}>
<Component {...pageProps} />
</Provider>
);
}
export default MyApp;
Using Redux in Components
Now that Redux is set up, you can use it in your components to manage global state.
Step 1: Connect Components to Redux
Connect your components to the Redux store using the connect
function from react-redux
:
// components/ReduxComponent.js
import { connect } from 'react-redux';
const ReduxComponent = ({ data, addData }) => {
return (
<div>
<h1>Redux Component</h1>
<ul>
{data.map((item, index) => (
<li key={index}>{item}</li>
))}
</ul>
<button onClick={() => addData('New Data')}>Add Data</button>
</div>
);
};
const mapStateToProps = (state) => ({
data: state.sample.data,
});
const mapDispatchToProps = (dispatch) => ({
addData: (data) => dispatch({ type: 'ADD_DATA', payload: data }),
});
export default connect(mapStateToProps, mapDispatchToProps)(ReduxComponent);
Conclusion
Integrating Redux into your Next.js application provides a centralized and efficient way to manage global state. By following these steps, you can set up a Redux store, create reducers, and connect your components to the store.
In the upcoming tutorials, we’ll explore more advanced topics such as real-time features, authentication with third-party providers, and continuous integration for Next.js applications.
Stay tuned for the next tutorial where we’ll dive into adding real-time functionality to your Next.js app using WebSocket.
Happy coding!