Adding Serverless Functions to Your Next.js App
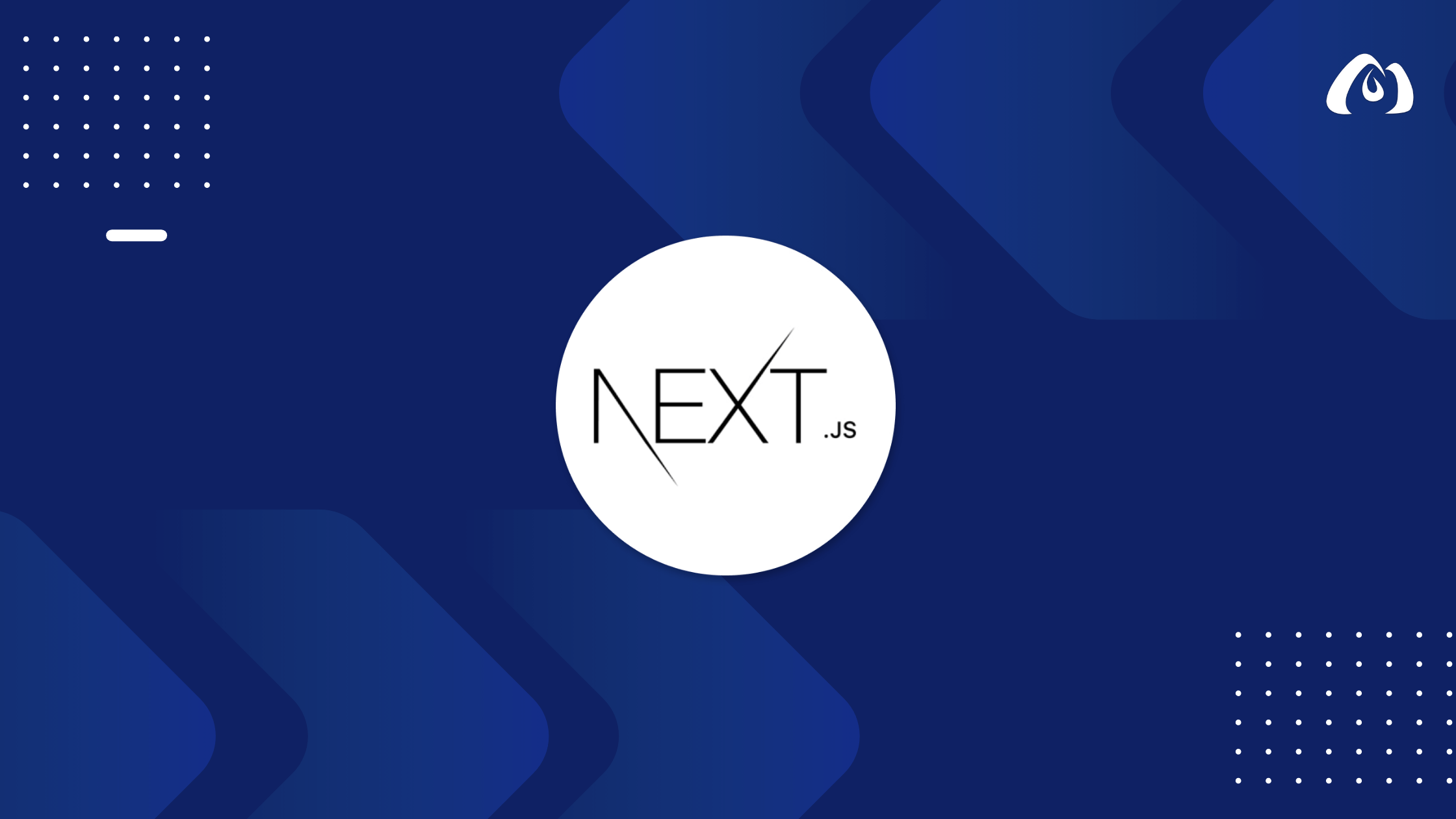
Serverless functions provide a convenient way to run backend logic without managing traditional servers. In this tutorial, we’ll explore how to add serverless functions to your Next.js application using the built-in API routes.
Creating a Serverless Function Permalink
Next.js allows you to create serverless functions by utilizing the pages/api
directory. Let’s create a simple example:
Step 1: Create a New API Route Permalink
Inside the pages/api
directory, create a new file named hello.js
:
// pages/api/hello.js
export default (req, res) => {
res.status(200).json({ message: 'Hello from the serverless function!' });
};
Step 2: Accessing the Serverless Function Permalink
You can now access this serverless function by making a request to /api/hello
in your Next.js app.
Passing Data to Serverless Functions Permalink
You can also pass data to serverless functions through query parameters or request bodies. Let’s modify our example to accept a name parameter:
Step 1: Update the API Route Permalink
// pages/api/greet/[name].js
export default (req, res) => {
const { name } = req.query;
res.status(200).json({ greeting: `Hello, ${name}!` });
};
Step 2: Accessing the Updated Serverless Function Permalink
Now, you can access this serverless function by making a request to /api/greet/John
to get a personalized greeting.
Conclusion Permalink
Integrating serverless functions into your Next.js application allows you to handle backend logic without the need for a separate server. Whether you’re fetching data, handling forms, or performing other backend tasks, serverless functions in Next.js provide a streamlined solution.
In the upcoming tutorials, we’ll explore more advanced topics, including real-time features, authentication with third-party providers, and continuous integration for Next.js applications.
Stay tuned for the next tutorial where we’ll dive into adding real-time functionality to your Next.js app using WebSocket.
Happy coding!