Real-Time Data with Next.js and WebSocket
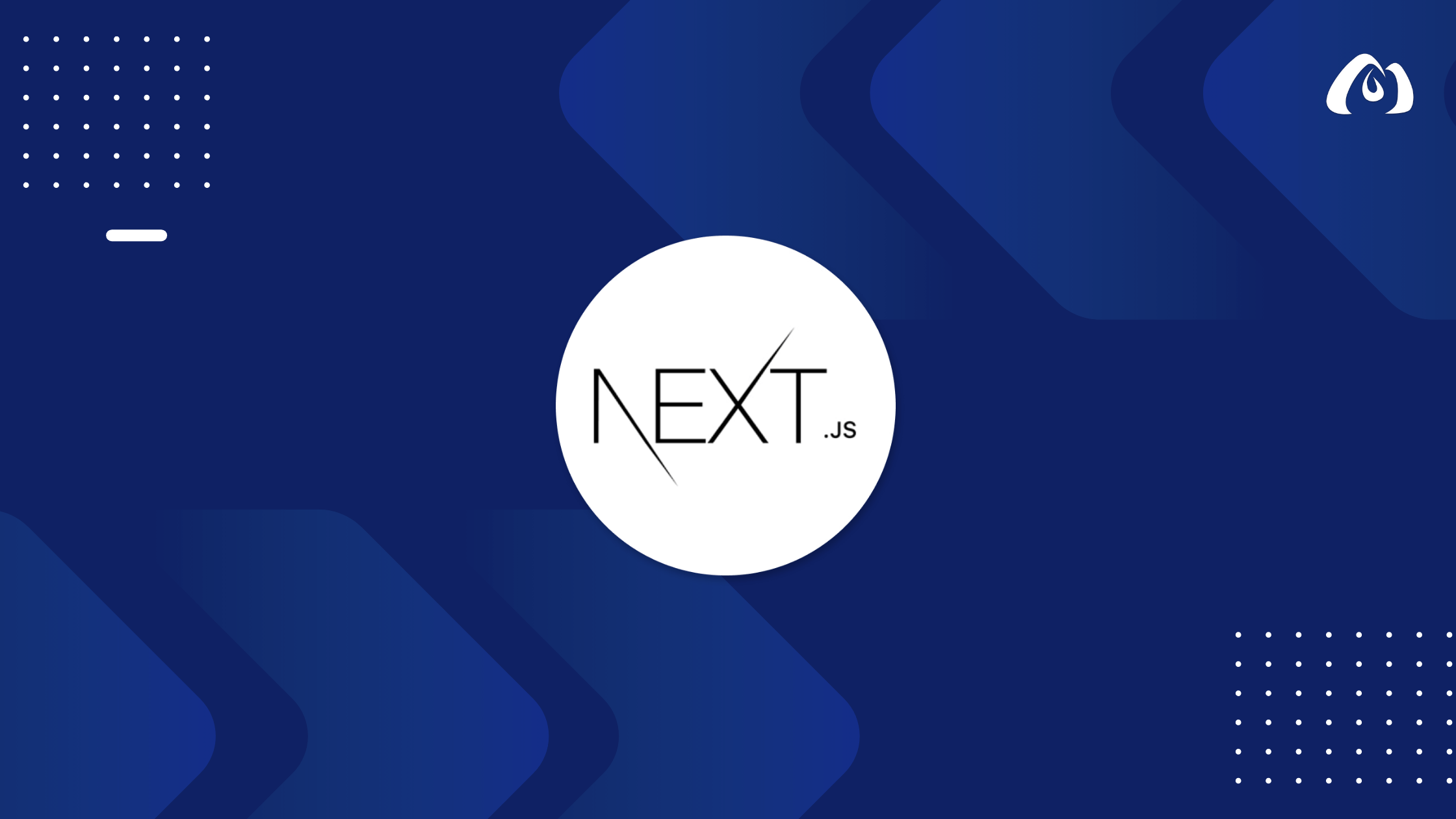
Real-time features provide users with instant updates and interactions, creating a dynamic and engaging user experience. In this tutorial, we’ll explore how to implement real-time data features in your Next.js application using WebSocket.
Understanding WebSocket
WebSocket is a communication protocol that enables bidirectional, real-time communication between a client and a server. Unlike traditional HTTP requests, WebSocket allows for continuous communication, making it ideal for real-time applications.
Setting Up WebSocket in Next.js
Step 1: Install Dependencies
Start by installing the ws
library, which provides WebSocket support:
npm install ws
Step 2: Create a WebSocket Server
Create a WebSocket server using Node.js. For simplicity, we’ll set up a basic server in a server.js
file:
// server.js
const WebSocket = require('ws');
const wss = new WebSocket.Server({ port: 3001 });
wss.on('connection', (ws) => {
ws.on('message', (message) => {
// Handle incoming messages
console.log(`Received message: ${message}`);
// Broadcast the message to all connected clients
wss.clients.forEach((client) => {
if (client !== ws && client.readyState === WebSocket.OPEN) {
client.send(message);
}
});
});
// Send a welcome message on connection
ws.send('Welcome to the WebSocket server!');
});
Step 3: Integrate WebSocket Server with Next.js
Modify your package.json
to run both the Next.js app and the WebSocket server. Update the "scripts"
section:
"scripts": {
"dev": "next dev",
"start": "node server.js",
"build": "next build",
"prod": "next start",
"websocket": "node server.js"
}
Now, you can run both the Next.js app and the WebSocket server with:
npm run dev
npm run websocket
Using WebSocket in Your Next.js Components
Step 1: Create a WebSocket Component
Create a new component, for example, WebSocketComponent.js
, that establishes a WebSocket connection:
// components/WebSocketComponent.js
import { useEffect, useState } from 'react';
const WebSocketComponent = () => {
const [messages, setMessages] = useState([]);
const [input, setInput] = useState('');
const ws = new WebSocket('ws://localhost:3001');
useEffect(() => {
ws.onopen = () => {
console.log('WebSocket connection opened');
};
ws.onmessage = (event) => {
// Handle incoming messages
setMessages((prevMessages) => [...prevMessages, event.data]);
};
ws.onclose = () => {
console.log('WebSocket connection closed');
};
// Clean up the WebSocket connection on component unmount
return () => {
ws.close();
};
}, []);
const sendMessage = () => {
if (input.trim() !== '') {
ws.send(input);
setInput('');
}
};
return (
<div>
<h1>WebSocket Chat</h1>
<div>
{messages.map((message, index) => (
<p key={index}>{message}</p>
))}
</div>
<input
type="text"
value={input}
onChange={(e) => setInput(e.target.value)}
placeholder="Type your message..."
/>
<button onClick={sendMessage}>Send</button>
</div>
);
};
export default WebSocketComponent;
Step 2: Use WebSocketComponent in Your Pages
Integrate the WebSocketComponent
into your Next.js pages or components where real-time chat functionality is desired.
// pages/index.js
import WebSocketComponent from '../components/WebSocketComponent';
const RealTimePage = () => {
return (
<div>
<h1>Real-Time Chat</h1>
<WebSocketComponent />
{/* Your page content goes here */}
</div>
);
};
export default RealTimePage;
Conclusion
Adding real-time data features to your Next.js application with WebSocket enhances user engagement and opens up possibilities for live updates, chat functionality, and more. By following these steps, you can implement WebSocket communication in your Next.js app and create dynamic, real-time experiences for your users.
In the upcoming tutorials, we’ll explore more advanced topics, including authentication with third-party providers and continuous integration for Next.js applications.
Stay tuned for the next tutorial where we’ll focus on adding authentication to your Next.js app using a third-party provider.
Happy coding and real-time development!