Handling Forms in Next.js
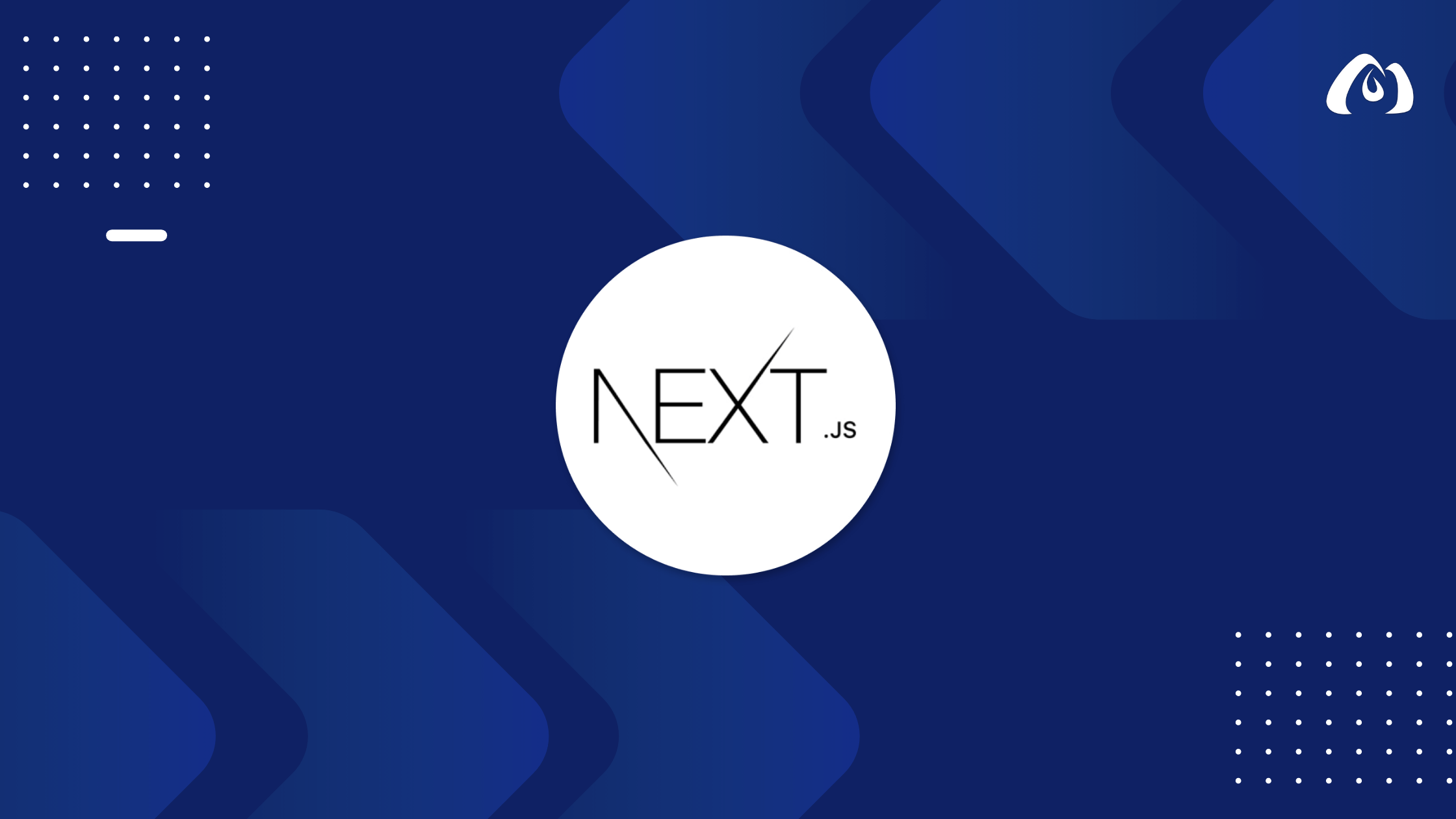
Forms are a fundamental part of web applications, enabling user interaction and data submission. In this tutorial, we’ll explore different strategies for handling forms in your Next.js application.
Basic Form Handling
Step 1: Create a Form Component
Start by creating a basic form component, for example, FormComponent.js
:
// components/FormComponent.js
import { useState } from 'react';
const FormComponent = () => {
const [formData, setFormData] = useState({
username: '',
email: '',
password: '',
});
const handleChange = (e) => {
const { name, value } = e.target;
setFormData((prevData) => ({
...prevData,
[name]: value,
}));
};
const handleSubmit = (e) => {
e.preventDefault();
// Handle form submission logic here
console.log('Form submitted:', formData);
};
return (
<form onSubmit={handleSubmit}>
<label>
Username:
<input type="text" name="username" value={formData.username} onChange={handleChange} />
</label>
<label>
Email:
<input type="email" name="email" value={formData.email} onChange={handleChange} />
</label>
<label>
Password:
<input type="password" name="password" value={formData.password} onChange={handleChange} />
</label>
<button type="submit">Submit</button>
</form>
);
};
export default FormComponent;
Step 2: Use the FormComponent in Your Pages
Integrate the FormComponent
into your Next.js pages or components where form functionality is desired.
// pages/index.js
import FormComponent from '../components/FormComponent';
const FormPage = () => {
return (
<div>
<h1>Form Handling in Next.js</h1>
<FormComponent />
{/* Your page content goes here */}
</div>
);
};
export default FormPage;
Using Form Libraries
Next.js provides flexibility in choosing form-handling approaches. Consider using popular form libraries such as react-hook-form
or formik
for more advanced form management, validation, and error handling.
Example with react-hook-form
npm install react-hook-form
// components/FormComponent.js
import { useForm } from 'react-hook-form';
const FormComponent = () => {
const { register, handleSubmit, formState } = useForm();
const onSubmit = (data) => {
// Handle form submission logic here
console.log('Form submitted:', data);
};
return (
<form onSubmit={handleSubmit(onSubmit)}>
<label>
Username:
<input {...register('username', { required: true })} />
</label>
<label>
Email:
<input {...register('email', { required: true })} />
</label>
<label>
Password:
<input {...register('password', { required: true })} />
</label>
<button type="submit" disabled={formState.isSubmitting}>
Submit
</button>
</form>
);
};
export default FormComponent;
Conclusion
Handling forms in your Next.js application involves basic form components and the option to use advanced form libraries based on your project requirements. By following these steps, you can effectively manage user input, validation, and form submissions in your Next.js app.
In the upcoming tutorials, we’ll explore more advanced topics, including image optimization, search functionality, and integration with external services.
Stay tuned for the next tutorial where we’ll focus on optimizing images in your Next.js application.
Happy coding and form handling in Next.js!