Building a Blog with Next.js and MDX
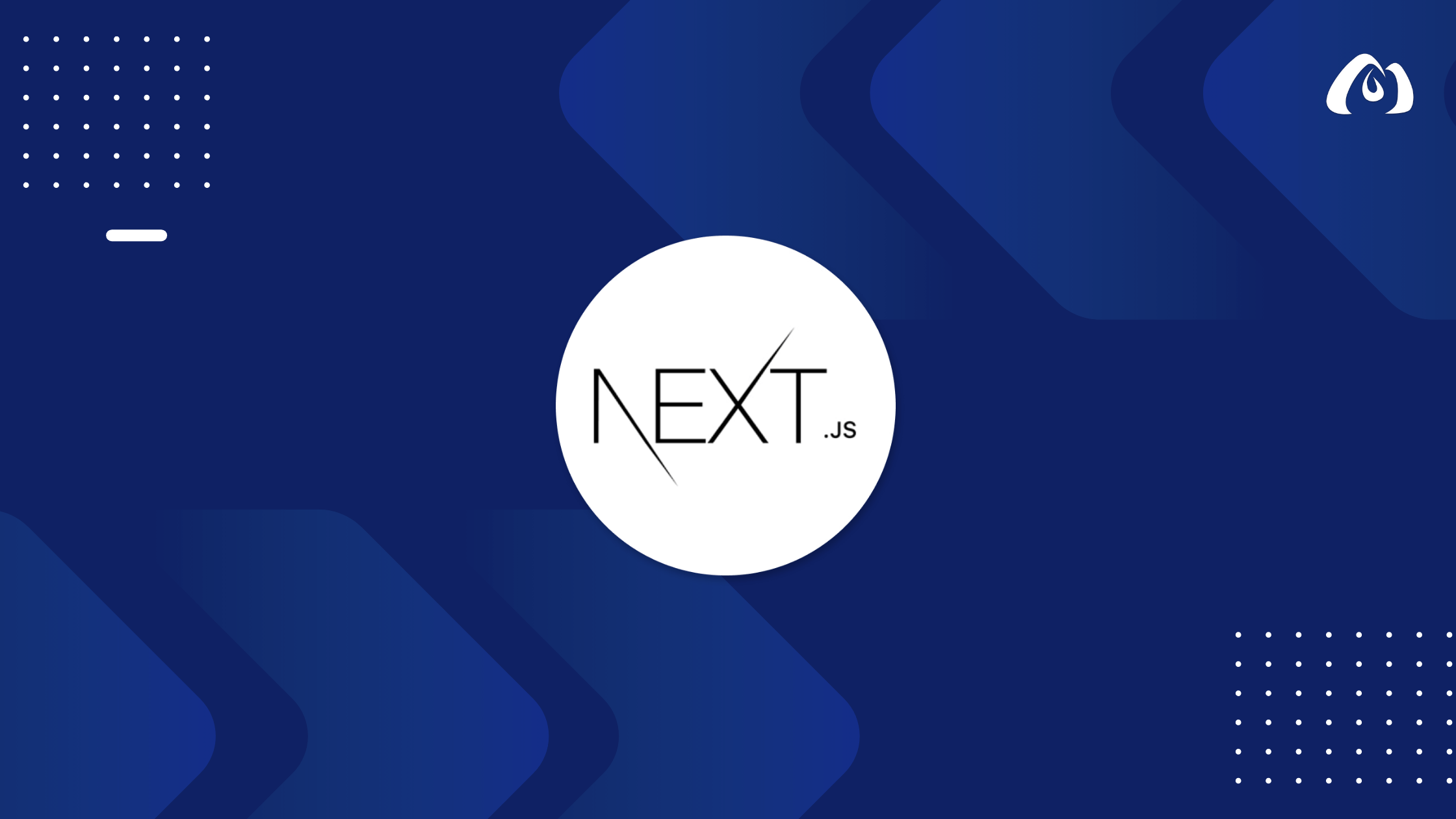
Creating a dynamic and flexible blog is a common use case for many websites. In this tutorial, we’ll explore how to build a blog with Next.js and MDX (Markdown for the component era) to enable easy content creation and customization.
Setting Up Next.js with MDX
Step 1: Install Dependencies
Install the necessary dependencies for Next.js and MDX:
npm install next react react-dom @next/mdx @mdx-js/loader
Step 2: Configure Next.js to Support MDX
Create a next.config.js
file in the root of your project:
// next.config.js
const withMDX = require('@next/mdx')();
module.exports = withMDX({
pageExtensions: ['js', 'jsx', 'md', 'mdx'],
});
Step 3: Create a Blog Folder
Create a folder named blog
in your project to store your blog posts in MDX format.
Creating MDX Blog Posts
Step 1: Create an MDX Blog Post
Inside the blog
folder, create an MDX file for your blog post, for example, 2023-01-19-Creating-a-Blog-with-Nextjs-and-MDX.mdx
:
---
layout: post
title: Creating a Blog with Next.js and MDX
image: /assets/img/blog/nextjs-mdx.png
---
# Creating a Blog with Next.js and MDX

Building a blog with Next.js and MDX provides a flexible and powerful way to manage your content. In this tutorial, we'll explore the steps to create a dynamic blog that supports Markdown-based content using MDX.
## Getting Started
### Step 1: Set Up Next.js with MDX
Follow the initial steps to install the necessary dependencies and configure Next.js to support MDX in your project.
### Step 2: Create a Blog Folder
Organize your blog posts by creating a dedicated folder, such as `blog`, to keep your MDX files.
## Writing Blog Content
### Step 1: Write in Markdown
Utilize the simplicity of Markdown to write your blog content. Include headings, lists, images, and other Markdown features to structure your posts.
### Step 2: Add Front Matter
Enhance your blog posts by adding front matter at the beginning of each MDX file. Front matter includes metadata such as the layout, title, and featured image.
```yaml
---
layout: post
title: Your Blog Post Title
image: /path/to/your/featured/image.png
---
Displaying Blog Posts
Step 1: Create a Blog Page
In your pages directory, create a page to display a list of blog posts. You can use the getFiles
utility to fetch the MDX files from the blog
folder.
// pages/blog/index.js
import { getFiles } from '../../utils/mdx';
const BlogPage = ({ posts }) => {
return (
<div>
<h1>Blog</h1>
<ul>
{posts.map((post) => (
<li key={post.filePath}>
<Link href={`/blog/${post.filePath.replace(/\.mdx?$/, '')}`}>
<a>{post.data.title}</a>
</Link>
</li>
))}
</ul>
</div>
);
};
export async function getStaticProps() {
const posts = await getFiles('blog');
return { props: { posts } };
}
export default BlogPage;
Step 2: Create a Blog Post Page
Create a page to display an individual blog post. Use the MDXProvider
to render the MDX content.
// pages/blog/[slug].js
import { MDXProvider } from '@mdx-js/react';
import { getFiles, getFileBySlug } from '../../utils/mdx';
const BlogPost = ({ mdxSource, frontMatter }) => {
return (
<div>
<h1>{frontMatter.title}</h1>
<MDXProvider>{mdxSource}</MDXProvider>
</div>
);
};
export async function getStaticPaths() {
const paths = await getFiles('blog');
return {
paths: paths.map((path) => ({
params: {
slug: path.replace(/\.mdx?$/, ''),
},
})),
fallback: false,
};
}
export async function getStaticProps({ params }) {
const post = await getFileBySlug('blog', params.slug);
return { props: post };
}
export default BlogPost;
Conclusion
Building a blog with Next.js and MDX empowers you to create dynamic, flexible, and content-rich websites. By following these steps, you can easily manage and customize your blog posts using Markdown and take advantage of the powerful features provided by Next.js and MDX.
In the upcoming tutorials, we’ll explore more advanced topics, including progressive image loading, real-time data with WebSocket, and integrating GraphQL.
Stay tuned for the next tutorial where we’ll focus on implementing progressive image loading in your Next.js application.
Happy coding and blogging with Next.js and MDX!