Progressive Image Loading in Next.js
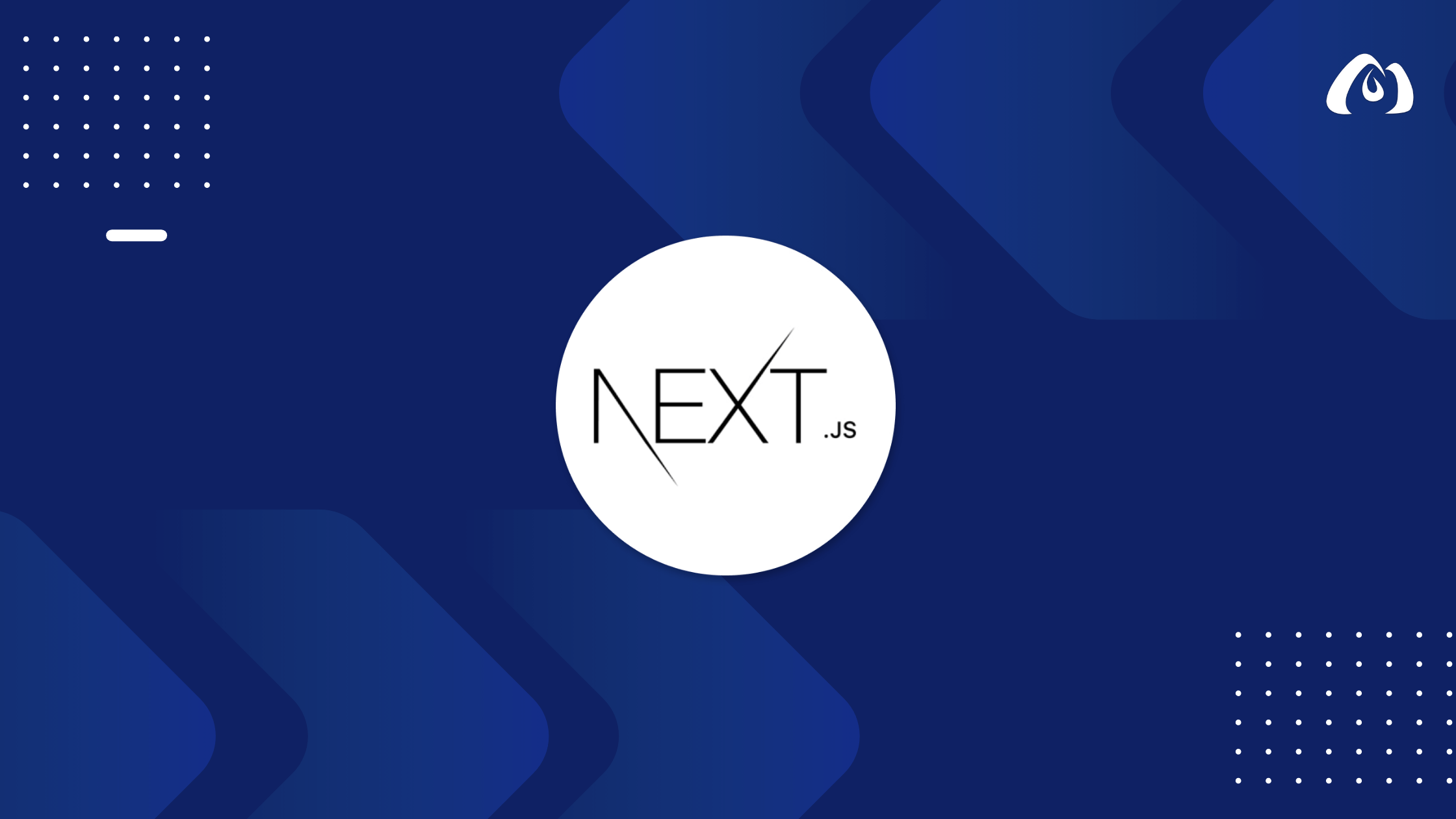
Enhancing user experience by optimizing image loading is crucial for web applications. In this tutorial, we’ll explore the implementation of progressive image loading in your Next.js application for faster page rendering.
Understanding Progressive Image Loading
Progressive image loading involves loading low-quality versions of images initially and gradually replacing them with higher-quality versions as the page loads. This technique improves perceived performance and provides a smoother user experience.
Implementing Progressive Image Loading in Next.js
Step 1: Install the lqip-loader
Install the lqip-loader
to generate low-quality base64-encoded placeholders for your images:
npm install lqip-loader
Step 2: Configure Webpack
Configure your Next.js project to use the lqip-loader
by updating the next.config.js
file:
// next.config.js
module.exports = {
webpack: (config) => {
config.module.rules.push({
test: /\.(jpe?g|png)$/i,
loader: 'lqip-loader',
options: {
base64: true,
palette: true,
},
});
return config;
},
};
Step 3: Update Image Components
Update your image components to use the low-quality placeholders initially and replace them with the higher-quality images as they load.
// components/ProgressiveImageComponent.js
import Image from 'next/image';
const ProgressiveImageComponent = () => {
return (
<div>
<h1>Progressive Image Loading in Next.js</h1>
<div>
<Image
src="/path/to/your/image.jpg"
alt="Description of the image"
width={800}
height={600}
placeholder="blur"
/>
</div>
{/* Your page content goes here */}
</div>
);
};
export default ProgressiveImageComponent;
Step 4: Customize Loading Behavior
Customize the loading behavior by adjusting the placeholder
property. Options include 'empty'
, 'blur'
, or a custom base64-encoded image.
// components/CustomizedLoadingImageComponent.js
import Image from 'next/image';
const CustomizedLoadingImageComponent = () => {
return (
<div>
<h1>Customized Loading Image in Next.js</h1>
<div>
<Image
src="/path/to/your/image.jpg"
alt="Description of the image"
width={800}
height={600}
placeholder={`data:image/svg+xml;base64,${yourBase64EncodedImage}`}
/>
</div>
{/* Your page content goes here */}
</div>
);
};
export default CustomizedLoadingImageComponent;
Conclusion
Implementing progressive image loading in your Next.js application is a valuable technique for optimizing page performance and providing a better user experience. By following these steps and customizing the loading behavior, you can control how images are initially displayed and replaced with higher-quality versions.
In the upcoming tutorials, we’ll explore more advanced topics, including real-time data with WebSocket and integrating GraphQL.
Stay tuned for the next tutorial where we’ll focus on adding real-time data features to your Next.js application using WebSocket.
Happy coding and progressive image loading in Next.js!