Real-Time Data with Next.js and WebSocket
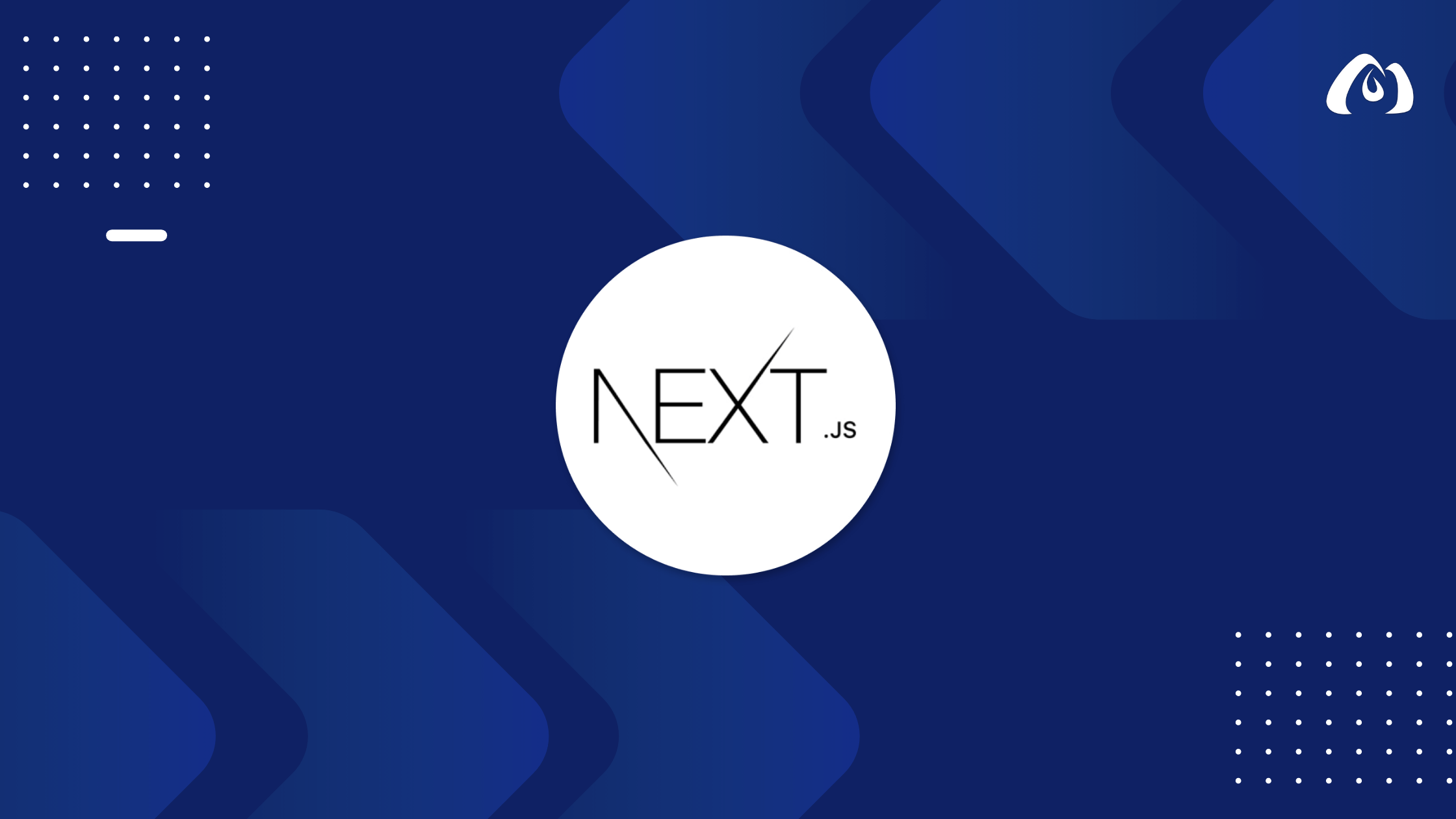
Implementing real-time features enhances the interactivity and engagement of your web application. In this tutorial, we’ll explore how to add real-time data capabilities to your Next.js application using WebSocket.
Understanding WebSocket
WebSocket is a communication protocol that enables bidirectional, real-time communication between clients and servers. It’s particularly useful for applications that require instant updates and notifications.
Implementing WebSocket in Next.js
Step 1: Install ws
Package
Install the ws
package, which is a simple WebSocket implementation for Node.js:
npm install ws
Step 2: Create a WebSocket Server
Create a WebSocket server in your Next.js project. For simplicity, let’s create a basic server file, for example, websocketServer.js
:
// websocketServer.js
const WebSocket = require('ws');
const wss = new WebSocket.Server({ noServer: true });
wss.on('connection', (ws) => {
ws.on('message', (message) => {
// Handle incoming messages from clients
console.log(`Received message: ${message}`);
});
// Send a welcome message to the connected client
ws.send('Welcome to the WebSocket server!');
});
module.exports = wss;
Step 3: Integrate WebSocket Server with Next.js
Integrate the WebSocket server with your Next.js application by updating the server.js
file:
// server.js
const http = require('http');
const { parse } = require('url');
const next = require('next');
const wss = require('./websocketServer'); // Import your WebSocket server
const dev = process.env.NODE_ENV !== 'production';
const app = next({ dev });
const handle = app.getRequestHandler();
app.prepare().then(() => {
const server = http.createServer((req, res) => {
const parsedUrl = parse(req.url, true);
handle(req, res, parsedUrl);
});
server.on('upgrade', (request, socket, head) => {
wss.handleUpgrade(request, socket, head, (ws) => {
wss.emit('connection', ws, request);
});
});
server.listen(3000, (err) => {
if (err) throw err;
console.log('> Ready on http://localhost:3000');
});
});
Step 4: Create a WebSocket Component
Create a WebSocket component in your Next.js project to handle real-time communication. For example, WebSocketComponent.js
:
// components/WebSocketComponent.js
import { useEffect } from 'react';
const WebSocketComponent = () => {
useEffect(() => {
const ws = new WebSocket('ws://localhost:3000');
ws.onopen = () => {
console.log('WebSocket connection established.');
};
ws.onmessage = (event) => {
// Handle incoming messages from the server
console.log(`Received from server: ${event.data}`);
};
return () => {
ws.close();
console.log('WebSocket connection closed.');
};
}, []);
return (
<div>
<h1>Real-Time Data with Next.js and WebSocket</h1>
{/* Your page content goes here */}
</div>
);
};
export default WebSocketComponent;
Step 5: Integrate WebSocket Component
Integrate the WebSocketComponent
into your Next.js pages or components where real-time data functionality is desired.
// pages/index.js
import WebSocketComponent from '../components/WebSocketComponent';
const IndexPage = () => {
return (
<div>
<WebSocketComponent />
{/* Your page content goes here */}
</div>
);
};
export default IndexPage;
Conclusion
Adding real-time data features to your Next.js application using WebSocket opens up opportunities for creating interactive and dynamic user experiences. By following these steps, you can establish a WebSocket connection and handle real-time communication between the server and clients.
In the upcoming tutorials, we’ll explore more advanced topics, including integrating GraphQL and adding authentication with Firebase.
Stay tuned for the next tutorial where we’ll focus on integrating GraphQL into your Next.js application.
Happy coding and real-time data with Next.js and WebSocket!