Map Integration with Mapbox and Next.js
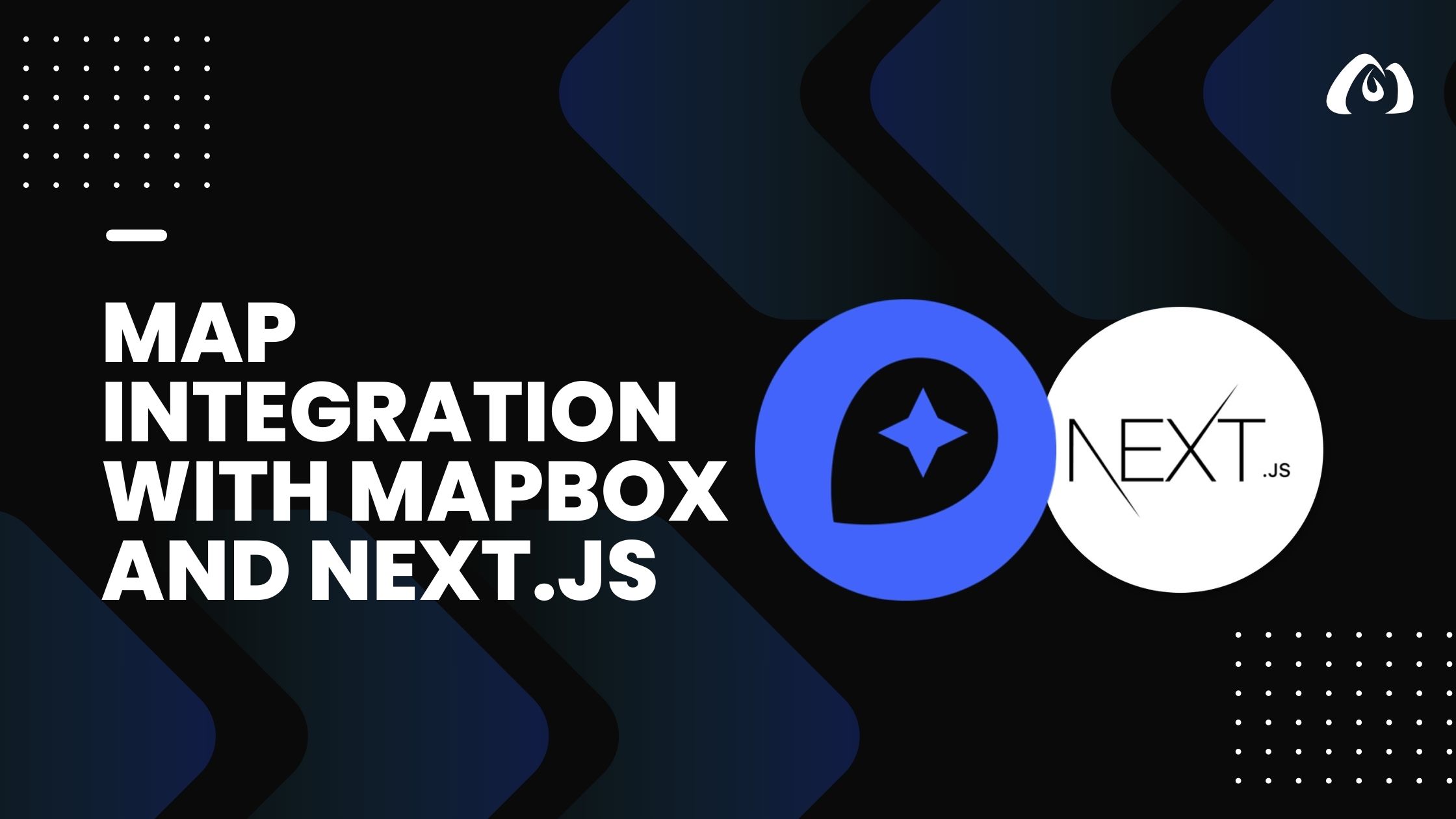
Adding maps to your Next.js applications can enhance user engagement and provide valuable location-based information. In this tutorial, we’ll explore how to integrate Mapbox, a popular mapping platform, into a Next.js project for seamless map integration.
Getting Started with Mapbox Permalink
Before we begin, make sure you have a Mapbox account and an access token. You can sign up for a Mapbox account at mapbox.com. Once you have your account, create a new access token from the Mapbox dashboard.
Integrating Mapbox in Next.js Permalink
1. Install the Mapbox React library Permalink
We’ll use the react-map-gl
library for integrating Mapbox into our Next.js application. Install it using npm or yarn:
npm install react-map-gl mapbox-gl
or
yarn add react-map-gl mapbox-gl
2. Create a Map Component Permalink
Create a new component for your map, for example, MapComponent.js
:
// components/MapComponent.js
import React from 'react';
import ReactMapGL from 'react-map-gl';
const MapComponent = () => {
const [viewport, setViewport] = React.useState({
latitude: 37.7577,
longitude: -122.4376,
zoom: 8,
});
return (
<ReactMapGL
{...viewport}
width="100%"
height="400px"
mapStyle="mapbox://styles/mapbox/streets-v11"
onViewportChange={(newViewport) => setViewport(newViewport)}
mapboxApiAccessToken={process.env.MAPBOX_ACCESS_TOKEN}
/>
);
};
export default MapComponent;
3. Set up Environment Variables Permalink
Store your Mapbox access token securely by adding it to your environment variables. Create a .env.local
file in the root of your Next.js project and add the following:
MAPBOX_ACCESS_TOKEN=your-mapbox-access-token
4. Integrate the Map Component Permalink
Now, integrate the MapComponent
into a page or layout where you want to display the map:
// pages/MapPage.js
import React from 'react';
import MapComponent from '../components/MapComponent';
const MapPage = () => {
return (
<div>
<h1>Map Integration with Mapbox and Next.js</h1>
<MapComponent />
</div>
);
};
export default MapPage;
Customize and Explore Permalink
You now have a basic Mapbox integration in your Next.js application. Customize the map by exploring the Mapbox Style Specification and adjusting the mapStyle
prop in the MapComponent
. Additionally, you can add markers, popups, and other interactive features to create a rich map experience for your users.
By integrating Mapbox with Next.js, you can provide users with valuable location-based information and create visually appealing maps that enhance the overall user experience.