Adding a Comment System with Discuss in Next.js
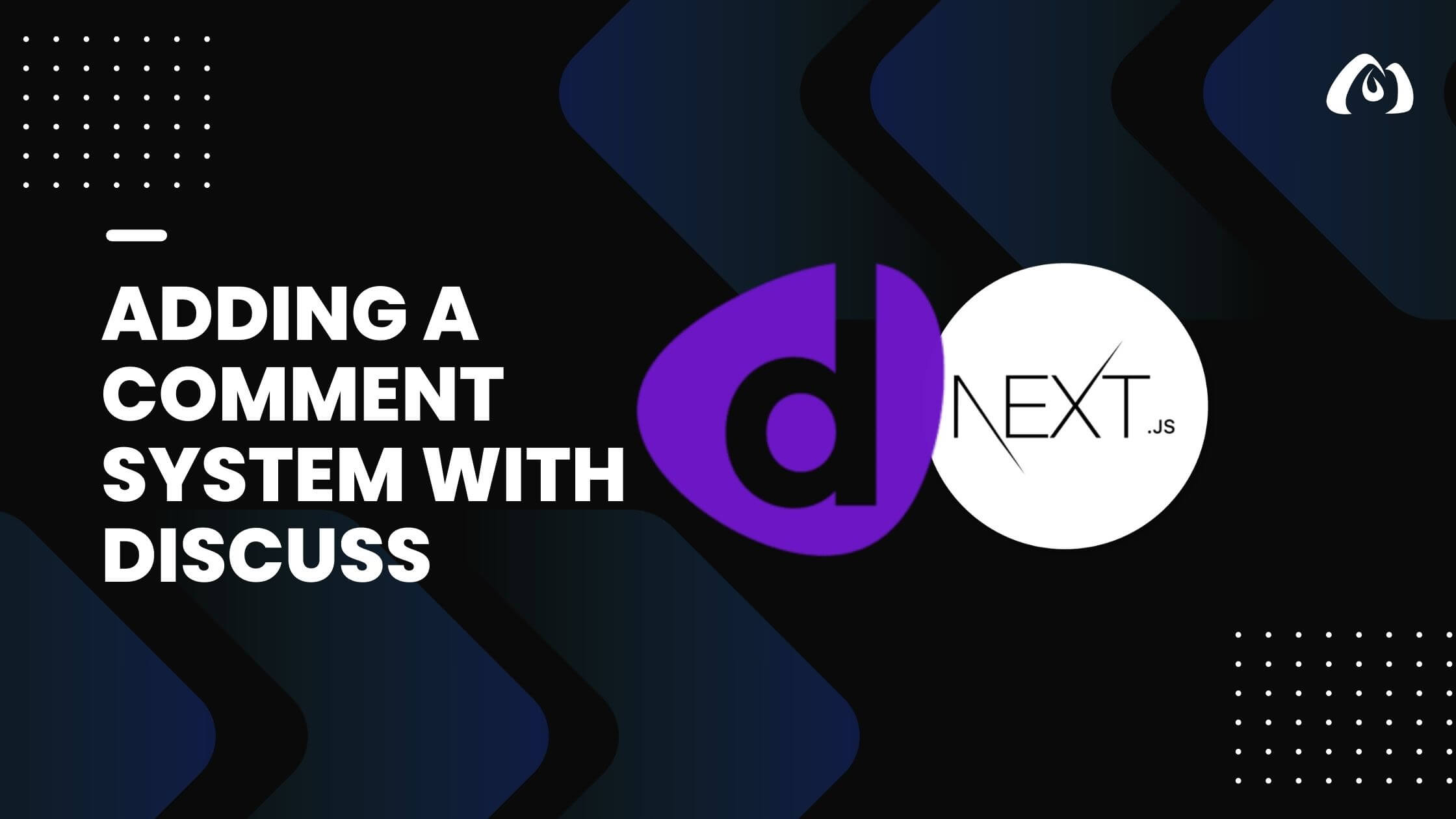
Engaging with your audience is crucial for building a community around your content. Adding a comment system to your Next.js blog can facilitate discussions and enhance user interaction. In this tutorial, we’ll explore how to integrate Discuss, a commenting platform, into a Next.js application for a seamless commenting experience.
Setting Up Discuss
Discuss is a third-party commenting platform that provides a simple and customizable solution for adding comments to your website. Here’s how you can set it up:
1. Create a Discuss Account
If you don’t have a Discuss account, sign up for one at discuss.io.
2. Create a New Site
After creating an account, log in to Discuss and create a new site. Follow the setup instructions, and you’ll obtain a unique Discuss shortname for your site.
Integrating Discuss in Next.js
Now, let’s integrate Discuss into your Next.js blog:
1. Install the Discuss React Component
Install the discuss-react
package, which provides a React component for embedding Discuss comments:
npm install discuss-react
or
yarn add discuss-react
2. Create a Comment Component
Create a new component named CommentComponent.js
that will encapsulate the Discuss comment system:
// components/CommentComponent.js
import React from 'react';
import { DiscussionEmbed } from 'discuss-react';
const CommentComponent = ({ discussShortname, discussConfig }) => {
return <DiscussionEmbed shortname={discussShortname} config={discussConfig} />;
};
export default CommentComponent;
3. Integrate the Comment Component
Now, integrate the CommentComponent
into your blog post page or layout where you want to display comments:
// pages/BlogPost.js
import React from 'react';
import CommentComponent from '../components/CommentComponent';
const BlogPost = () => {
return (
<div>
{/* Your blog post content */}
<h1>Adding a Comment System with Discuss in Next.js</h1>
<p>This is your blog post content...</p>
{/* Include the CommentComponent at the end of the post */}
<CommentComponent
discussShortname="your-discuss-shortname"
discussConfig=
/>
</div>
);
};
export default BlogPost;
Make sure to replace "your-discuss-shortname"
, "your-unique-post-id"
, and "Your Blog Post Title"
with your Discuss shortname, a unique identifier for the post, and your actual blog post title.
Customize and Moderate
Discuss provides various customization options and moderation features through its dashboard. Explore the settings to tailor the comment system to your website’s design and moderation needs.
Adding a comment system with Discuss enhances the interactivity of your Next.js blog, fostering community engagement and providing a platform for discussions around your content.