10 mini python projects
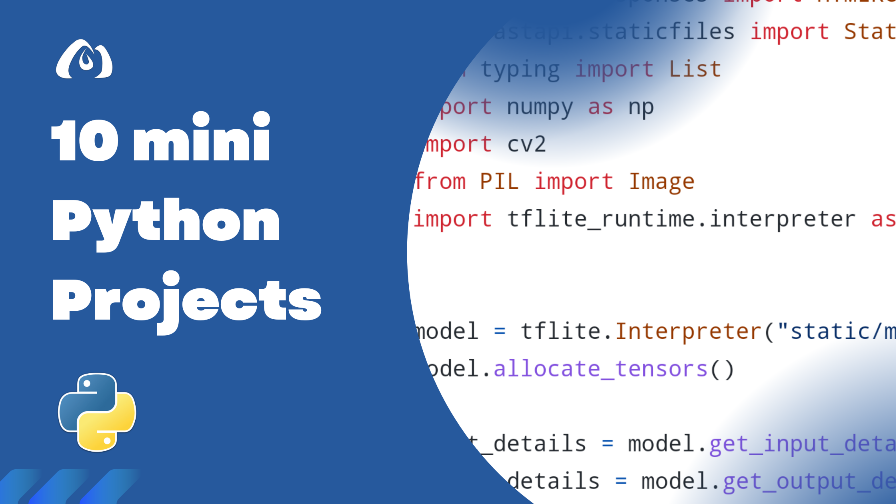
Python has been in the top 10 popular programming languages for a long time, as the community of Python programmers has grown a lot due to its easy syntax and library support. In this article, I will introduce you to 10 amazing Python projects with source code solved and explained for free. You can also goto to my github for more.
Python Projects with Source Code Permalink
Python Projects For Beginners: If you’re a newbie to Python where you’ve just learned lists, tuples, dictionaries, and some basic Python modules like the random module, here are some Python projects with source code for beginners for you:
1. Acronyms Generator
user_input = str(input("Enter a Phrase: "))
text = user_input.split()
a = " "
for i in text:
a = a+str(i[0]).upper()
print(a)
accronyms-generator.py
2. Email stripper
email = input("Enter Your Email: ").strip()
username = email[:email.index("@")]
domain_name = email[email.index("@")+1:]
format_ = (f"Your username is '{username}' and your domain is 'www.{domain_name}'")
print(format_)
email-stripper.py
3. Password Generator
import random
passlen = int(input("enter the length of password"))
s="abcdefghijklmnopqrstuvwxyz01234567890ABCDEFGHIJKLMNOPQRSTUVWXYZ!@#$%^&*()?"
p = "".join(random.sample(s,passlen ))
print(p)
password-generator.py
4. QR Code generator
import pyqrcode
from pyqrcode import QRCode
# String which represent the QR code
s = "https://youtube.com/channel/UC1rmAY_kabt5DlZX05PSNqQ"
# Generate QR code
url = pyqrcode.create(s)
# Create and save the png file naming "myqr.png"
url.svg("myyoutube.svg", scale = 8)
qr-code-generator.py
5. Quiz game
def check_guess(guess, answer):
global score
still_guessing = True
attempt = 0
while still_guessing and attempt < 3:
if guess.lower() == answer.lower():
print("Correct Answer")
score = score + 1
still_guessing = False
else:
if attempt < 2:
guess = input("Sorry Wrong Answer, try again")
attempt = attempt + 1
if attempt == 3:
print("The Correct answer is ",answer )
score = 0
print("Guess the Animal")
guess1 = input("Which bear lives at the North Pole? ")
check_guess(guess1, "polar bear")
guess2 = input("Which is the fastest land animal? ")
check_guess(guess2, "Cheetah")
guess3 = input("Which is the larget animal? ")
check_guess(guess3, "Blue Whale")
print("Your Score is "+ str(score))
quiz-game.py
6. Story Generator
import random
when = ['A few years ago', 'Yesterday', 'Last night', 'A long time ago','On 20th Jan']
who = ['a rabbit', 'an elephant', 'a mouse', 'a turtle','a cat']
name = ['Ali', 'Miriam','daniel', 'Hoouk', 'Starwalker']
residence = ['Barcelona','India', 'Germany', 'Venice', 'England']
went = ['cinema', 'university','seminar', 'school', 'laundry']
happened = ['made a lot of friends','Eats a burger', 'found a secret key', 'solved a mistery', 'wrote a book']
print(random.choice(when) + ', ' + random.choice(who) + ' that lived in ' + random.choice(residence) + ', went to the ' + random.choice(went) + ' and ' + random.choice(happened))
story-generator.py
7. Temperature Converter
def convert(s):
f = float(s)
c = (f - 32) * 5/9
return round(c,0)
temp = input('Please enter temperature in Fahrenheits:\n')
print(str(convert(temp))+"°C")
temparature-converter.py
8. OTP System
import os
import math
import random
import smtplib
digits="0123456789"
OTP=""
for i in range(6):
OTP+=digits[math.floor(random.random()*10)]
otp = OTP + " is your OTP"
msg= otp
s = smtplib.SMTP('smtp.gmail.com', 587)
s.starttls()
s.login("Your Gmail Account", "You app password")
emailid = input("Enter your email: ")
s.sendmail('&&&&&&&&&&&',emailid,msg)
a = input("Enter Your OTP >>: ")
if a == OTP:
print("Verified")
else:
print("Please Check your OTP again")
otp-system.py