Creating Your First FastAPI Endpoint
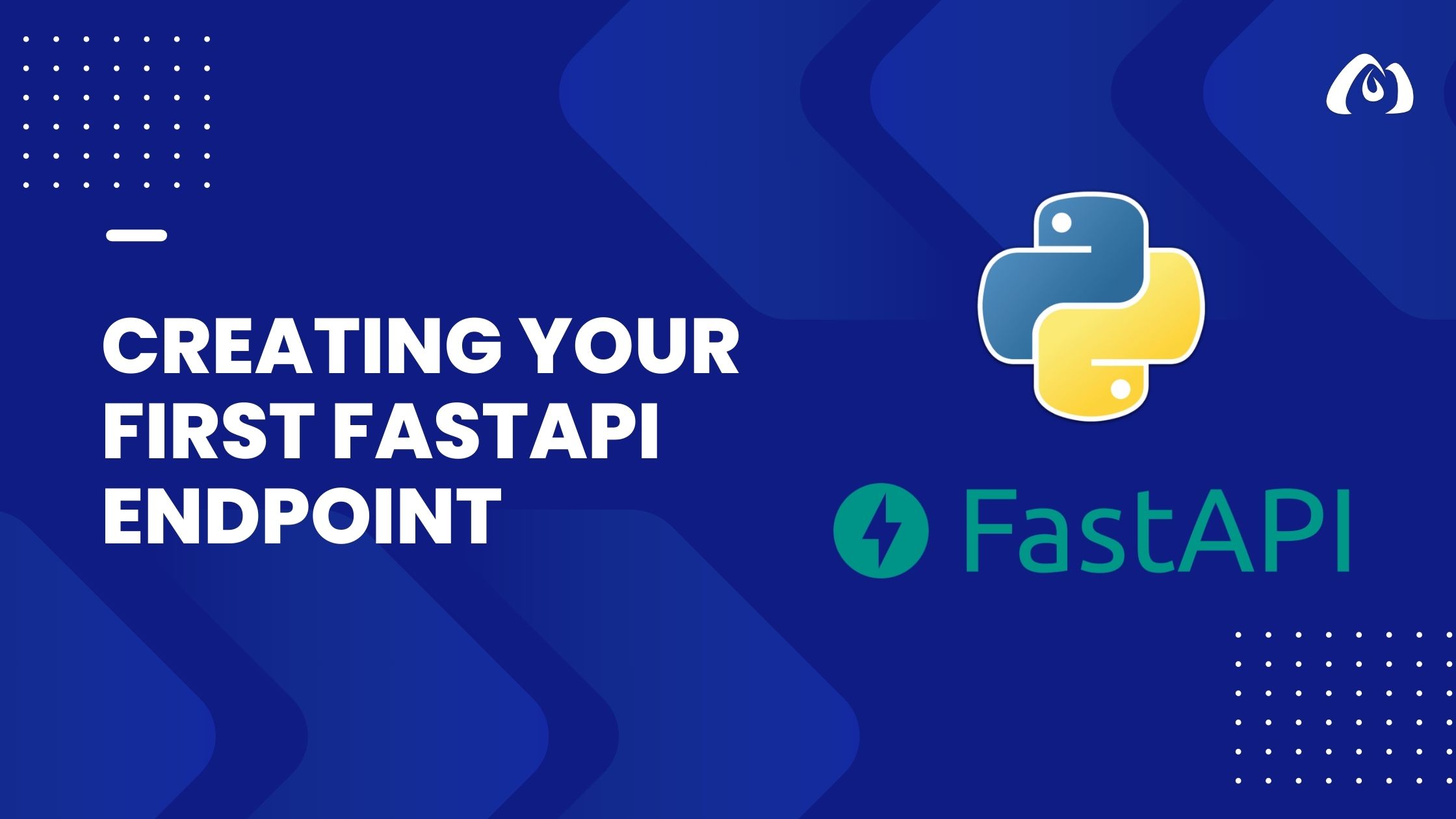
In the previous tutorial, we introduced FastAPI and built a basic application with a single route. Now, let’s take the next step and create our first FastAPI endpoint. We’ll explore how to define endpoints, handle HTTP methods, and return data in various formats.
Defining Endpoints in FastAPI
In FastAPI, endpoints are defined using decorators to declare the path and the HTTP method. Let’s create a simple endpoint that returns a greeting. Open your main.py
file and modify it as follows:
# main.py
from fastapi import FastAPI
app = FastAPI()
@app.get("/")
def read_root():
return {"Hello": "World"}
@app.get("/greet/{name}")
def greet(name: str):
return {"message": f"Hello, {name}!"}
In this example, we’ve added a new endpoint /greet/{name}
, which takes a name
parameter. The @app.get
decorator indicates that this endpoint responds to HTTP GET requests.
Running Your FastAPI Application
To see our new endpoint in action, let’s run the FastAPI application with Uvicorn:
uvicorn main:app --reload
Visit http://127.0.0.1:8000 in your browser or a tool like Swagger. You’ll find the new /greet/{name}
endpoint in the documentation.
Interacting with the Endpoint
To interact with our new endpoint, simply append a name to the URL. For example, http://127.0.0.1:8000/greet/John will return a JSON response:
{"message": "Hello, John!"}
FastAPI automatically validates the input parameters based on their type annotations and generates documentation accordingly.
Handling Query Parameters
Let’s enhance our endpoint by adding an optional query parameter. Modify the greet
function in main.py
as follows:
# main.py
from fastapi import FastAPI
app = FastAPI()
@app.get("/")
def read_root():
return {"Hello": "World"}
@app.get("/greet/{name}")
def greet(name: str, greeting: str = "Hello"):
return {"message": f"{greeting}, {name}!"}
Now, the greet
endpoint accepts an additional query parameter greeting
, with a default value of “Hello.” You can test this by visiting http://127.0.0.1:8000/greet/Mary?greeting=Hola.
Conclusion
Congratulations! You’ve successfully created your first FastAPI endpoint. In this tutorial, we explored defining routes, handling parameters, and interacting with your API through the automatic documentation provided by FastAPI.
In the next tutorial, we’ll delve deeper into handling request parameters, both in the path and as query parameters. Stay tuned for more exciting FastAPI features!